Ask Kathleen
Visual Studio 2010 Tips: Debug WCF Services in Silverlight Apps
- By Doug Gregory
- 11/01/2010
Q. In previous Silverlight applications, I created my Windows Communication Foundation (WCF) services by adding a new WCF service item to my Web Project and filling in the code behind. This has lead to problems testing and debugging my service configurations. Is there a better way to add a WCF service?
A. There are many ways to create a WCF service, but the method I'll describe makes it easier to troubleshoot problems and take advantage of the lightweight WCF configuration options offered in Visual Studio 2010.
The simplest way to add a service for your Silverlight application is to add a WCF Service item to the Web application project of your Silverlight solution. When you add a service named TestServiceCB, Visual Studio creates an interface with a sample service definition:
[ServiceContract]
public interface ITestServiceCB
{
[OperationContract]
void DoWork();
}
In addition, Visual Studio adds a TestServiceCB.svc file with the following code behind, which implements the service interface:
public class TestServiceCB : ITestServiceCB {
public void DoWork() {
}
}
If you select TestServiceCB.svc and choose "View In Browser" from the context menu, the browser opens to the URL localhost:55778/TestServiceCB.svc and displays an information page about the service. Note that the port -- 55778 in this URL -- will differ for your environment.
This solution works for simple services, but may not be suitable for more complicated services involving business and data-access tiers that may require integration and unit testing. It also makes it very difficult to deploy the same service using a different host than your Web application. For these situations, a project structure shown in Figure 2 may make more sense.
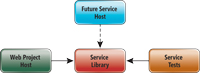 [Click on image for larger view.] |
Figure 2. A separate service library for your WCF services allows you to host them in a variety of ways and facilitates testing your configurations. This isn't what Visual Studio does by default, but it's not hard to build. |
With this approach, all interfaces and classes used to implement the service are contained in a separate service library project. The Web Project still hosts the service, calling on the classes in the service library as needed. One or more Test Projects are created to directly test the classes in the Service Library, thereby reducing the likelihood of problems and giving you a way to debug that bypasses the client. This is particularly important in the Silverlight and WCF world, where all exceptions in your service return generic SOAP faults with little or no diagnostic information by default.
To create this structure, add a new WCF Service Library to your Silverlight solution. By default, Visual Studio adds an interface named IService1.cs and service class called Service1.cs to your Service Library project. These are analogous to the Interface and code-behind classes created when you add a WCF service directly to your Web application. You should change the name of the interface and the service class to reflect the name of your service. As an example, a service called TestService might contain the following ITestService interface:
[ServiceContract]
public interface ITestService
{
[OperationContract]
DateInformation GetFutureDate(ref ServiceContext context,
int futureDays);
}
And a corresponding service class to implement the service:
public class TestService : ITestService {
public DateInformation GetFutureDate(
ref ServiceContext context, int futureDays) {
return null;
}
}
In order to host your service in your Silverlight application's corresponding Web application, you'll need to add a reference to the Service Library project and create a file named TestService.svc. You must first add the file as a text file and change its prefix. Add the following markup to the TestService.svc file:
<%@ ServiceHost Language="C#" Debug="true" Service = "MyTest-
Service.TestService" %>
You need to configure your service in the Web application's Web.config file. The WCF team put significant effort into reducing the complexity of WCF configurations in the Microsoft .NET Framework 4 by using default values for most bindings. This means you technically don't need to add any configuration information for the service, but for development, some configuration overrides may be useful. Add the following to your Web.config:
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior>
<serviceMetadata httpGetEnabled="true"/>
<serviceDebug includeExceptionDetailInFaults="false"/>
</behavior>
</serviceBehaviors>
</behaviors>
<serviceHostingEnvironment multipleSiteBindingsEnabled="true" />
</system.serviceModel>
The service Metadata tag tells WCF to publish metadata that's used when you add a service reference in your Silverlight application. On your local machine, Visual Studio will automatically assign an address to your service. For deployment to production, you'll need to add additional configuration information to specify the actual URL for your service. Note that Visual Studio creates a configuration section in the App.config of your Service Library project, but WCF ignores that configuration information.
Testing Service Configurations
You're now ready to test that your service has been wired up correctly. First, test that your configuration file is set up properly by highlighting the SVC file in Solution Explorer, right-clicking and selecting "View in a Browser." The browser should display an informational page about your service. Note the automatically generated URL.
To test that your service is actually callable, Visual Studio supplies the WCF Test Client tool. To start the tool, open a Visual Studio command prompt and type the command wcftestclient. When the tool window pops up, add your service by selecting File | Add Service and supplying the URL you noted when you opened the SVC file in the browser. From the Test Client you can invoke each of your service methods with test data and see the results. The WCF Test Client is shown in Figure 3.
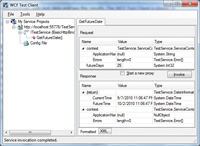 [Click on image for larger view.] |
Figure 3. The WCF Test Client provides a way to verify that a service has been wired up properly. The Request and Response sections on the right provide a way to run smoke tests on the selected operation. |
At this point, you've completed server-side wiring for your service. You can build out your service by adding operations, business and data-access layers as you see fit. I always add a service test project to test the Service Library. This testing usually pays off later by helping me avoid time-consuming and frustrating issues when attempting to diagnose service problems through the Silverlight client application.
About the Author
Doug Gregory, a consulting Enterprise Architect with Spitfire Group LLC, is a guest columnist for Kathleen Dollard this month. He explains how Visual Studio 2010 ASP.NET MVC 2 works with client-side behavior using jQuery, and shows you how to add a DatePicker control.