Code Focused
An EF Code First Tutorial
Code First frees you up from the chore of creating databases for your project. Here's a primer on how to do it.
Entity Framework has been the latest buzzword in the Microsoft Development circle since the release of Visual Studio 2010. With the release of Entity Framework 4.1 in April 2011, EF became even more useful with the introduction of Code First. EF is an ORM (Object Relational Mapping) framework that abstracts the details of the database structure from the application through the use of a model.
The first step to working with a database is to create the database. EF allows for this to happen in three ways:
- Database First: Importing an existing database into the EF Model
- Model First: Creating the model and using it to generate the database
- Code First: Using code classes to generate the database
This tutorial focuses on No. 3. Like many technical topics, the best way to explain Code First is to demonstrate it.
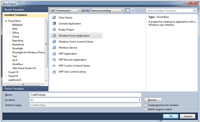 [Click on image for larger view.] |
Figure 1. Creating the new project. |
1. Install EF 4.1 from http://bit.ly/oFL43y
2. Create the Application
- Start Visual Studio 2010
- Click File/New/Project…
- Select Windows from the left pane and Windows Forms Application, as shown in Figure 1. Note: in this example, I'll utilize the Visual Basic Templates in the left pane; a similar template is available in Visual C#.
- Enter "CodeFirstApp" as the name and click "OK".
3. Create the Model
Next, I'm going to create a separate class library (Figure 2), called DataAccess. This will be used for creating the initial database structure and subsequent data access later in the demo. Utilizing a separate project for these tasks will adhere to the concept of SoC (Separation of Concerns).
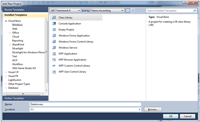 [Click on image for larger view.] |
Figure 2. Creating the class library |
For additional punctuality, I'm going to rename Form1.vb to frmMain.vb and Class1.vb to DataBaseStruct.vb, as shown in Figure 3.
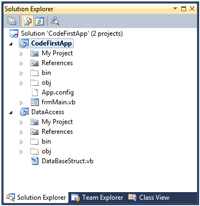 [Click on image for larger view.] |
Figure 3. Renaming Form1.vb and Class1.vb. |
References
In addition to the default references loaded in the project template, I added a reference to "EntityFramework" (EntityFramework.dll) to both projects. I also added a reference to the "DataAccess" project from the "CodeFirstApp" project.
Define the Database
With everything in place, I can start creating the database structure in the DataBaseStruct.vb. Code First uses classes and properties to identify tables and columns, respectively. For this example, I'll be using a simple database structure called "Transportation" with two tables: Vehicles and VehicleTypes, having a one-to-one relationship.
First I define the table structure for the Vehicle table by defining it as a class with four properties:
- VehicleID As Int32
- Make As String
- Model As String
- VehicleType As VehicleType
EF recognized that the first property contains ID in the name and immediately set it as the Primary Key for that table. Since it's a Primary Key, it was automatically set to be Not Null. Properties Make and Model were defined as a String type without a size; therefore, EF will set them to nvarchar(max), null in the database.
The last property is called VehicleType, and is used as a foreign key to the VehicleType table. Note this field is of type "VehicleType" (the structure of the table it points to). The VehicleType (singular) will be used to build the VehicleTypes (plural) table.
The same logic applies for building the VehicleTypes table. Three properties are defined in this table:
- VehicleTypeID As Int32
- Name As String
- Description As String
With all the properties defined in their respective classes, they can now be declared as collections and used to define the table structures:
Public Class TransportationDB
Inherits DbContext
Public Property Vehicles() As DbSet(Of Vehicle)
Public Property VehicleTypes() As DbSet(Of VehicleType)
End Class
Notice the tables are defined using the DbSet collections. DbSet was introduced in EF 4.1 as a wrapper object around ObjectSet. This object is utilized for all CRUD operations against the table. Also in EF 4.1, Microsoft introducted DbContext. This object is a wrapper for the ObjectContext object, abstracting away the complexities; it's the preferred method for interacting with the database. Because of this, the class that defines the database structure will inherit the DbContext class. Both DbSet and DbContext are defined in the System.Data.Entity namespace.
Figure 4 shows a complete listing of the DataBaseStruct.vb.
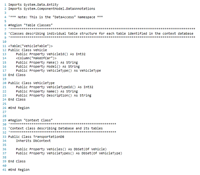 [Click on image for larger view.] |
Figure 4. DataBaseStruct.vb. |
4. Implement and Populate the Database
At this stage, the Database structure is fully defined as a "Code First" model, but hasn't been implemented on the server. For this example I'll utilize a simple form and place the logic in the "Create Database" button event handler, which can be seen in Figure 5.
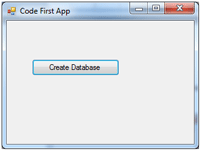 [Click on image for larger view.] |
Figure 5. Implementing Code First on the server through a button. |
To deploy and populate the database, simply instantiate the classes created, populate the data fields and execute SaveChanges() on the Database context object. The complete code from frmMain.VB is shown in Figure 6.
 [Click on image for larger view.] |
Figure 6. frmMain.vb. |
The VehicleTypes table will be referenced by the Vehicle table, so it needs to be populated first. Note the first call to context.SaveChanges() on line 20 will create the database and insert the VehicleType record. Moving to line 23, the Vehicle object is instantiated and associated with the previously inserted record.
By default, DbContext will create the database on localhost\SQLExpress. The default database name will follow the fully qualified name (Namespace.ObjectName) of the derived DbContext object. In this example, the database name is "DataAccess.TransportationDB".
 [Click on image for larger view.] |
Figure 7. The resulting database. |
Looking at SQL Server Management Studio, Figure 7, the database, tables and columns are implemented per the object names defined in DataBaseStruct.vb.
Data Annotations
If you look closer at the database implementation in comparison to the classes defined in DataBaseStruct.vb, you'll see the outcome of using data annotations on the class. Using <Table("VehicleTable")> on the Vehicle class allowed the table to be called "VehicleTable" in the database, but was still accessible by referencing the Vehicle class.
Similarly, <Column("MakeOfCar")> allowed the column in the database to be called "MakeOfCar" while it was referenced in code as "Make". These attributes and many others are found in the namespace System.ComponentModel.DataAnnotations.
Advantages of Code First
Code First has a number of benefits. First, developers are no longer delayed by not having a database. You can now create the database structure and start coding, with everything maintained in the same solution. Second, there's no auto-generated code; a developer has full control of each of the classes. Lastly, everything is kept simple in the data access layer because there's no .EDMX model to update or maintain.
About the Author
Sam Nasr has been a software developer since 1995, focusing mostly on Microsoft technologies. Having achieved multiple certifications from Microsoft (MCAD, MCTS(MOSS), and MCT), Sam develops, teaches, and tours the country to present various topics in .Net Framework. He is also actively involved with the Cleveland C#/VB.Net User Group, where he has been the group leader since 2003. In addition, he also started the Cleveland WPF Users Group in June 2009, and the Cleveland .Net Study Group in August 2009, and is the INETA mentor for Ohio. When not coding, Sam loves spending time with his family and friends or volunteering at his local church. He can be reached by email at [email protected].