C# Corner
Roslyn CTP Custom Refactoring
Learn how to extend Visual Studio 2010 by creating code issue providers with the Roslyn CTP.
Have you ever used Visual Studio and thought of a clever or useful refactoring that wasn't part of the tooling? Well now, with the Rosyln CTP--Microsoft's test version of a C# compiler that exposes public APIs--you can put that idea into fruition.
I'll show you how to add a custom code issue provider and action to Visual Studio. It will analyze a target method and give you an option to make the method static, if it isn't using any class members. In addition, the method in question will be displayed with a red underline to provide a visual cue of the code issue.
Create a Code Issue
First, I'll go over how to create a custom code issue and then show you how to provide the code action to correct the issue. To get started, launch Visual Studio 2010 with SP1 and create a new Code Issue project as shown in Figure 1. The template is located under the Roslyn category.
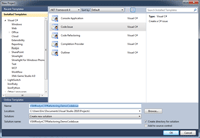 [Click on image for larger view.] |
Figure 1. Creating a new Code Issue Project |
Open up the CodeIssueProvider class file. You should see a few methods named GetIssues that return IEnumerable<CodeIssue>. The template creates a sample code issue. It displays x contains the letter 'a', where x is a namespace, class or method name, which contains an 'a' character in its identifier. You'll notice three overrides for the GetIssues method, which take one of three types of syntax: CommonSyntaxNode, CommonSyntaxToken and CommonSyntaxTrivia object. Each method is used to provide a code issue for its type of element. The type of provider for the code issue is declared through a Managed Extensibility Framework (MEF) export attribute on the class. The template sample uses an ExportSyntaxNodeCodeIssueProvider attribute to declare that the provider is only concerned with syntax nodes.
Now, I'll implement a custom code issue that will analyze a method to see if it can be made static. First, I'll update the MEF export for the code issue to only analyze method declarations.
[ExportSyntaxNodeCodeIssueProvider("VSMRolynCTP.DemoCodeIssue",
LanguageNames.CSharp, typeof(MethodDeclarationSyntax))]
Next, I'll update the GetIssues method to determine if the method can be made static.
public IEnumerable GetIssues(IDocument document, CommonSyntaxNode node,
CancellationToken cancellationToken)
{
I then cast the CommonSyntaxNode to a MethodDeclarationSyntax node, and check if the method is already static, by seeing if any of its descendents contain the static keyword. If the method is already static, then there's no issue, so I return an empty IEnumerable.
var methodDeclaration = (MethodDeclarationSyntax)node;
if (methodDeclaration.DescendentTokens().Any(x => x.Kind ==
SyntaxKind.StaticKeyword))
{
yield return null;
}
else
{
If the method is not already static, I get all of its identifiers to see if they are class members through an IsClassMember helper function.
var semanticModel = document.GetSemanticModel(cancellationToken);
IEnumerable memberIdentifiers = from m in
methodDeclaration.DescendentNodes()
where m as IdentifierNameSyntax
!= null
&&
IsClassMember(cancellationToken,semanticModel,m as ExpressionSyntax)
select m as IdentifierNameSyntax;
If the method doesn't contain any class member identifiers, then it can be made into a static method. In this case, I yield return a new CodeIssue that has an information severity, stating the method can be made static.
if (!memberIdentifiers.Any())
{
yield return new CodeIssue(CodeIssue.Severity.Info, methodDeclaration.Span,
String.Format("{0} can be made static.", methodDeclaration));
}
}
}
The IsClassMember helper method analyzes a given ExpressSyntax to determine if its symbol was defined at a class level. I use a semantic model to retrieve the semantic information for the given syntax. If the symbol exists, is not static, and was defined in a non-local scope, it's a member identifier. The full IsClassMember code is shown below:
private static bool IsClassMember(CancellationToken cancellationToken, ISemanticModel
semanticModel, ExpressionSyntax syntax)
{
bool isMember = false;
var memberInfo = semanticModel.GetSemanticInfo(syntax, cancellationToken);
if (memberInfo.Symbol != null && !memberInfo.Symbol.IsStatic &&
memberInfo.Symbol.OriginalDefinition.Kind != CommonSymbolKind.Local)
{
isMember = true;
}
return isMember;
}
By now, you should have the find static method code issue, shown in Listing 1.
If you run the code issue now, you'll notice that a new instance of Visual Studio 2010 has loaded. Create a new Console Application to verify that the code issue is working as shown in Figure 2.
 [Click on image for larger view.] |
Figure 2. New Console Application for Code Issue |
If you go to Tools, then Extensions Manager, you'll notice that the custom code issue has been installed (Figure 3).
 [Click on image for larger view.] |
Figure 3. Installed Custom Code Issue |
Now, you should notice that if you create some methods, any that don't contain access to a class member will be highlighted in red via the installed custom code issue provider (Figure 4).
 [Click on image for larger view.] |
Figure 4. Code Issue in Action |
Identify a Static Method
So far, I've only shown half of the story. I've created a custom code issue, now it's time to take action. The custom code action will allow the user to make the method in question static.
To start, create a new class for the project named StaticMethodCodeAction.First, you need to add using statements for the necessary Roslyn CTP namespaces as follows:
using Roslyn.Compilers;
using Roslyn.Compilers.CSharp;
using Roslyn.Services;
using Roslyn.Services.Editor;
Next, create a constructor that takes an ICodeActionEditFactory, IDocument and MethodDeclarationSyntax, that'll be called from the code issue provider. The following code creates the StaticMethodCodeAction constructor:
ICodeActionEditFactory _editFactory;
IDocument _document;
MethodDeclarationSyntax _methodDeclaration;
public StaticMethodCodeAction(ICodeActionEditFactory editFactory,
IDocument document, MethodDeclarationSyntax methodDeclaration)
{
_editFactory = editFactory;
_document = document;
_methodDeclaration = methodDeclaration;
}
Now, modify the description property to state "Make method static" as follows:
public string Description
{
get { return "Make method static"; }
}
Now, it's time to implement the heart of the code action--the GetEdit method. The GetEdit method will create a new syntax tree, with the method updated to include the static keyword.
public ICodeActionEdit GetEdit(System.Threading.CancellationToken cancellationT
{
To start, you need to get the first token and any leading trivia. Trivia can be anything that's not significant to the meaning of the expression, such as whitespace or comments.
var firstToken = _methodDeclaration.GetFirstToken();
var firstTokenTrivia = firstToken.LeadingTrivia;
Next, create a new static keyword token with the same leading trivia as the original first token.
var formattedMethodDeclaration = _methodDeclaration.ReplaceToken(
firstToken, firstToken.WithLeadingTrivia(Syntax.TriviaList()));
var staticToken = Syntax.Token(firstTokenTrivia, SyntaxKind.StaticKeyword);
Then, you create a set of modifiers that includes the new static-keyword token.
var newModifiers = Syntax.TokenList(
new[] { staticToken }.Concat(formattedMethodDeclaration.Modifiers));
Now, you create a new method-declaration syntax that contains the new formatted method declaration.
var newMethodDeclaration = formattedMethodDeclaration.Update(
formattedMethodDeclaration.Attributes,
newModifiers,
formattedMethodDeclaration.ReturnType,
formattedMethodDeclaration.ExplicitInterfaceSpecifierOpt,
formattedMethodDeclaration.Identifier,
formattedMethodDeclaration.TypeParameterListOpt,
formattedMethodDeclaration.ParameterList,
formattedMethodDeclaration.ConstraintClauses,
formattedMethodDeclaration.BodyOpt,
formattedMethodDeclaration.SemicolonTokenOpt);
Add an annotation to the new method declaration to indicate the result of the change.
var formattedLocalDeclaration = CodeActionAnnotations.FormattingAnnotation
.AddAnnotationTo(newMethodDeclaration);
Next, replace the old method declaration with the new formatted declaration in the document's syntax tree.
var tree = (SyntaxTree)_document.GetSyntaxTree();
var newRoot = tree.Root.ReplaceNode(_methodDeclaration,
formattedLocalDeclaration);
Lastly, you return a new code action that transforms the syntax tree to include the new method declaration through use of the CreateTreeTransormEdit factory method on the CodeActionEditFactory instance.
return _editFactory.CreateTreeTransformEdit(_document.Project.Solution,
syntaxTree, newRoot, cancellationToken: cancellationToken);
}
See Listing 2 for the full GetEdit method implementation.
Next, update the Icon to return null.
public System.Windows.Media.ImageSource Icon
{
get { return null; }
}
Lastly, update the CodeIssueProvider class's GetIssues method to return the StaticMethodCodeAction with the code issue, which allows the user to make the method static.
if (!memberIdentifiers.Any())
{
yield return new CodeIssue(CodeIssue.Severity.Info, methodDeclaration.Span,
String.Format("{0} can be made static.", methodDeclaration),
new StaticMethodCodeAction(editFactory, document, methodDeclaration));
}
Now, you can run the custom code issue provider, shown in Figure 5, and use the newly created code action!
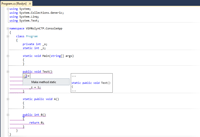 [Click on image for larger view.] |
Figure 5. Code Issue and Action Completed |
The Roslyn CTP is only a preview, but it already shines. This article has shown you how to extend Visual Studio with a custom code-issue provider that identifies a static method. You can take this concept much further and start increasing your Visual Studio productivity. As a bonus, you'll gain a greater understanding of the C# language
About the Author
Eric Vogel is a Senior Software Developer for Red Cedar Solutions Group in Okemos, Michigan. He is the president of the Greater Lansing User Group for .NET. Eric enjoys learning about software architecture and craftsmanship, and is always looking for ways to create more robust and testable applications. Contact him at [email protected].