Wahlin on .NET
Creating a Silverlight-Enabled WCF Service
As Dan shows, creating Silverlight-enabled services is as easy as picking the proper template in Visual Studio 2008 and adding a little code.
Silverlight is capable of consuming data from a variety of sources including REST APIs, ASMX services, Windows Communication Foundation (WCF) services and other standards-compliant services. In my previous article, I discussed how Silverlight's WebClient class could be used to communicate with a REST API and retrieve data. The ability to access data from REST APIs is nice any time you'd like to create mash-up applications that aggregate and display data from a variety of other sites and services.
However, for many applications you'll want to retrieve and display your own data. Several different options exist for this including ASMX services, ADO.NET Data Services and WCF services. Let's take a look at how to create a Silverlight-enabled WCF service using Visual Studio 2008.
Creating a WCF ServiceWCF provides several different ways for clients to bind to a service. You can use wsHttpBinding, netTcpBinding, basicHttpBinding and many others depending on the needs of client applications. In cases where Silverlight 2 clients will be calling WCF services, you'll need to use basicHttpBinding since Silverlight 2 doesn't support the encryption classes necessary to encrypt and decrypt SOAP messages using WS-Security standards. If data needs to be secured between the client and the server, SSL (https) can be used.
Although you can create a WCF service and then manually change the binding in the service's configuration file, the easiest way to get started creating WCF services is to use the Silverlight-enabled WCF Service template in the Add New Item dialog of Visual Studio 2008. By using this template, all of the necessary configuration options will automatically be created to allow for a basicHttpBinding. Figure 1 shows the template.
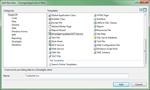 [Click on image for larger view.] |
Figure 1. Once the Silverlight tools are installed for Visual Studio you'll see the Silverlight-enabled WCF Service option. |
You can add the WCF service to the test Web project that's added by default when you create a new Silverlight 2 project or to another Web project. Once it's added, a service class will be created for you as shown in Listing 1:
[ServiceContract(Namespace = "")]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class CustomerService
{
[OperationContract]
public void DoWork()
{
// Add your operation implementation here
return;
}
// Add more operations here and mark them with [OperationContract]
}
Listing 1: The default class generated by the Silverlight-enabled WCF Service template.
The basicHttpBinding required by Silverlight 2 is automatically added to web.config so that you don't have to manually change any of the configuration settings. (Note that Silverlight 3 now supports binary message encoding by default which provides even better performance.)
Once the WCF service class is added into your Web project, you can write methods to return the necessary objects from the service and decorate the methods with the WCF OperationContract attribute. This attribute marks them as service operations so that they can be consumed by a Silverlight client. Listing 2 shows an example of adding a service operation called GetCustomer that returns a Customer object. Although the code returns a Customer object directly, you could of course add the necessary code to call a database and return dynamic data:
[ServiceContract(Namespace = "http://www.TheWahlinGroup.com")]
[AspNetCompatibilityRequirements(RequirementsMode =
AspNetCompatibilityRequirementsMode.Allowed)]
public class CustomerService
{
[OperationContract]
public Customer GetCustomer(int id)
{
return new Customer { FirstName = "John", LastName = "Doe", Zip = 85244 };
}
// Add more operations here and mark them with [OperationContract]
}
public class Customer
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Zip { get; set; }
}
Listing 2: Adding a WCF operation named GetCustomer that returns a Customer object.
Creating Silverlight-enabled services is as easy as picking the proper template in Visual Studio 2008 and adding a little code. In the next article, I'll walk through the process of consuming a WCF service in Silverlight 2.
About the Author
Dan Wahlin (Microsoft MVP for ASP.NET and XML Web Services) is the founder of The Wahlin Group which specializes in .NET and SharePoint onsite, online and video training and consulting solutions. Dan also founded the XML for ASP.NET Developers Web site, which focuses on using ASP.NET, XML, AJAX, Silverlight and Web Services in Microsoft's .NET platform. He's also on the INETA Speaker's Bureau and speaks at conferences and user groups around the world. Dan has written several books on .NET including "Professional Silverlight 2 for ASP.NET Developers," "Professional ASP.NET 3.5 AJAX, ASP.NET 2.0 MVP Hacks and Tips," and "XML for ASP.NET Developers." Read Dan's blog here.