Code Focused
Experiencing C# from a VB Developer's Perspective
On VB author Joe Kunk talks about his impression of C#.
I've tried learning Spanish, French and Japanese. Based on the success of those efforts, I think it is safe to say that if I really want to be multi-lingual, it will have to be with computer languages. A little over two years ago I left my job as a .NET developer for an organization which coded solely in Visual Basic and joined a very dynamic consulting firm with an extensive client list. As a consultant, I work in the language required by the client and thus have spent significant time working in C# over the last two years, in addition to supporting our Visual Basic clients.
In this article I share my thoughts on C# from a Visual Basic developer's perspective. My hope is that this article will help C# developers interact more effectively with developers from a Visual Basic background and also to alleviate some of the trepidation that Visual Basic developers may feel as they approach C#. I know from experience that a discussion on the relative merits of computer languages can quickly turn vitriolic. Before that happens, I want to clearly state that this article simply describes my experiences and reactions to those experiences and is not meant to present one language as better than the other.
Leverage what you already know
I clearly remember the most striking thing about my first days working in C# was a sense of amazement at how quickly I became productive. Not expert, but certainly productive. Both C# and Visual Basic utilize the .NET Common Language Runtime (CLR) and my existing knowledge of the CLR gave me a comfort level while in the C# editor with the ability to use many familiar classes, methods, and events.
Certainly there are syntax differences between common elements of C# and Visual Basic that express essentially the same logic. The syntax of "for (int i = 0; i < 11;="" i++)"="" looks="" a="" bit="" strange="" to="" someone="" accustomed="" to="" "for="" index="1" to="" 10".="">
Adapting to the new syntax was relatively easy. Typing a keyword like "if" and pressing tab twice stubs out the syntax and I was not forced to remember the syntactical nuances of these statements.
There are good resources that relate Visual Basic to C# syntax such as the extensive and well maintained chart VB.NET and C# Comparison produced by Dr. Frank McCown of the Harding University Computer Science Department. For those interested there is an equally useful chart (Java and C# Comparison) for those interested.
A great resource for understanding the equivalent C# source code is the free tool Reflector available from RedGate Software. When unsure of the C# syntax, I write a snippet of the desired functionality in Visual Basic, compile it, open it in Reflector and disassemble it with the C# option. For example, I wrote the following Fibonacci sequence calculation for F10 in Visual Basic as shown in Listing 1. It displays the number 55.
Public Sub Fibonacci()
Dim fibonacci = 0
For i = 1 To 10
fibonacci += i
Next
Dim msg = "Fibonacci Sequence of 10th iteration is : "
MessageBox.Show(msg + fibonacci.ToString())
End Sub
Listing 1. Fibonacci sequence calculation to 10th place in Visual Basic .Net
Opening the assembly in Reflector and choosing the C# option, I see the screen shown in Figure 1. It is interesting to note that the compiler eliminated the definition of the msg string variable in favor of an in-line definition within the MessageBox.Show method. For a quick conversion of a code snippet, you can take advantage of the the on-line developerFusion code converters.
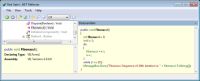 [Click on image for larger view.] |
Figure 1. Visual Basic source code of Listing 1 disassembled in C# syntax |
Some things just work differently
The one feature that scared me the most about working in C# is its case-sensitivity. Like most Visual Basic developers, I am very accustomed to and happy with the fact that I rarely have to think about letter case outside of string literals. Typing a variable name in all lowercase and then seeing to automatically convert to the proper mixed case as I had defined it is a great verification that I got the name right when I typed it.
The case-sensitivity turned out to be not much of an issue. IntelliSense works great in C# and corrects my casing when I accept its suggestion. I really don't spend much more time thinking about case-sensitivity in C# and I have become fond of the practice of naming local variables the same as properties except with a leading lowercase letter.
My fingers needed to learn C#. There are many things that I don't have to type in Visual Basic and I must remember to type in C#. A quick list:
- Typing a semicolon after each statement. Visual Basic eliminated the underscore as the line continuation character in Visual Basic 2010. Is the semicolon in C# next?
- Typing the ( ) after a method and [ ] for indexes.
- Typing "\r\n" instead of vbCrLf.
- Typing both the opening and closing brackets of a statement body if I don't use the template.
- Typing the Select clause for each LINQ statement. Don't you know that I just want the iterator?
- Type == for equality. I have forgotten this and accidently assigned a value in-line. Oops!
- Typing var in my iterator definitions such as foreach (var J in collectionList).
- Namespaces are more explicit. I have to include "System." as a prefix more often.
- Typing " identifier = new ()" instead of the shorter "Dim identifier as new ".
- Typing ? and ?? for ternary and null coalescing operators respectively.
Some things I like better in C#
Here is a quick list of the things I like better about C#:
- The ability to easily type an expression. Example: "(int)localVariable = someExpression;".
- The conciseness of the ? and ?? operators.
- Partial interfaces.
- The clarity of the out and ref parameter modifiers versus ByRef and ByVal.
- IntelliSense seems to work more intuitively.
Some things I like better in Visual Basic
Here is a quick list of the things I like better about Visual Basic:
- The productivity of the capabilities available via the My.* namespace.
- Automatically generated statement closing blocks.
- Better edit-and-continue capabilities.
- Auto-wireup of events via the Handles clause
- The With construct to reduce typing when specifying numerous properties on a class.
- XML Literals.
- Inline date declarations using the #1/1/2010# syntax.
- Better background compilation and more responsive error window updates.
Finally, consider that Visual Basic is often vertically more concise as it does not use an extra line for the statement block start. Consider this in C#:
if (condition)
{
// statements for true
}
else
{
// statements for false
}
And then consider this in Visual Basic:
IF condition Then 'statements for true Else 'statements for false End If
Conclusion
In general I find it easy to switch back and forth between C# and Visual Basic. Both are first class languages in their own right. There are features that I like better in each language, but within a few minutes of working in the "other" language, I am comfortable once again.
Remember that the question of C# versus Visual Basic is not an either-or proposition. Knowing each language well makes you a more valuable developer and I strongly recommend that you become proficient in both and write multi-language solutions that allow you to do your tasks in the language that is most productive for that particular task. Consider going beyond C# or Visual Basic and learn other .NET languages such as F#, IronRuby and IronPython. I am confident you will be glad you did.
About the Author
Joe Kunk is a Microsoft MVP in Visual Basic, three-time president of the Greater Lansing User Group for .NET, and developer for Dart Container Corporation of Mason, Michigan. He's been developing software for over 30 years and has worked in the education, government, financial and manufacturing industries. Kunk's co-authored the book "Professional DevExpress ASP.NET Controls" (Wrox Programmer to Programmer, 2009). He can be reached via email at [email protected].