Mono for Android
How-to: Create an Android Support Package
Google has a set of libraries that allow older versions of Android to get support for newer APIs. Mono for Android provides full support.
Much has been made about fragmentation of the Android platform. The truth is that the Android platform is not as fragmented as developers think. Google provides a set of libraries that allow older versions of Android to get support for newer APIs. In this article, I'll create a version of the Star Trek navigation application (from my previous column) that runs on Android 2.x to 4.x for handsets and tablets.
The Android Support Package is a set of static libraries with additional APIs that can be used by an application. These libraries back port some newer APIs to older versions of Android. At the current time, there are two versions of the Support Package.
The v4 Support Package is designed to bring Fragment and other support to Android 1.6 (API Level 4) and later. If a program needs to use some new features and run on Android 2.2, this is the library that it needs to use. Our sample application will use the v4 Support Package.
The v13 Support Package is similar in concept to the v4 Support Package. This package requires Android 3.2 (API Level 13) or later. It has some underlying implementation changes.
Getting Started
There's a multi-step process in setting up an application to use the Android Support Package. To get a project setup to use the v4 Support Package, complete the following steps.
First, download the Android Support Library in the Android SDK, as shown in Figure 1.
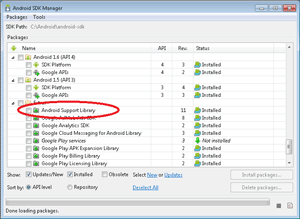 [Click on image for larger view.] |
Figure 1. Install the Android Support Library package in the Android SDK. |
Next, create an Android Application in Visual Studio (or MonoDevelop). In the project settings of the project, set the Minimum Android to target value to Android 1.6 (or a later version) as shown in Figure 2.
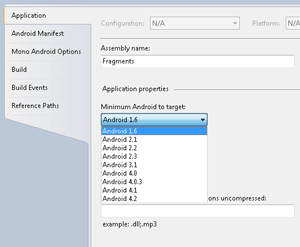 [Click on image for larger view.] |
Figure 2. Set the Minimum Android to target value to Android 1.6. |
Then add a reference in the project to Mono.Android.Support.v4, as shown in Figure 3.
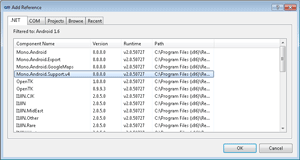 [Click on image for larger view.] |
Figure 3. Add a reference to Mono.Android.Support.v4. |
The next step is to add in the Android-Support-v4.jar. The library is located in the extras directory of the Android SDK. Place this library into the directory called "SupportLib" in the project, and change its Build Action to AndroidJavaLibrary.
Now that the application is set up, it will be possible to use many of the APIs provided by Fragments. The addition of the v4 Support Package does not "magically" turn on support for Fragments in an existing application. The Support Package provides similar APIs. Noticeable differences include the following:
- Activity. An Android application that hosts Fragments must inherit from Android.App.FragmentActivity. Previously, an Activity would inherit from Android.App.Activity.
- Namespaces. Classes that previously inherited from Android.App.Fragment must now inherit from Android.Support.v4.Fragment.
- FragmentManager. With Android.App.Activity, there's a FragmentManager property. The Android.App.FragmentActivity provides the SupportingFragmentManager property. This property is used to obtain a reference to the FragmentManager.
Aside from these differences, the APIs are fairly consistent. Now, let’s move to some code.
Screen Layouts
The first thing to look at in the application is the activity code shown in Listing 1, which starts the application. In this Activity1.cs code, the application will load the user interface that's defined in the Resource.Layout.Main resource. The project has a regular-sized layout for a handset and a tablet -specific keyboard for larger devices. These layouts are stored within the Layout and Layout-Large directory of the Resources folder. It's important to notice how screen sizes and densities affect the display. Android, before 3.2 (API Level 13) supports the following sizes:
- Small. A small screen is up to approximately 3.7 inches diagonal.
- Normal. A normal screen is between approximately 3.5 and 4.5 inches diagonal.
- Large. A large screen is between approximately 4.1 and 7 inches diagonal.
- XLarge. An extra large screen is approximately 7 inches diagonal and up.
Android uses the screen size of the device and the density of the display to determine the appropriate layout resource to display within the application. A discussion of building a device independent screen layout is beyond the scope of this article; however, it's important to understand the basics. For this application, notice that there are two directories containing layouts. One is the Layout directory, which contains the layout files used for a handset display. The Layout-large directory contains the layout files for a larger device, in this case a tablet.
Let’s take a look at the Main Layouts for a handset. In the following code, the TitlesFragment is loaded. The Fragment is the Fragment defined in the TitlesFragment.cs file.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<fragment class="com.wallym.sample.fragmentexample.TitlesFragment"
android:id="@+id/titles_fragment"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
</LinearLayout>
The Main Layout for a tablet is shown in Listing 2. The Fragment defined in the TitlesFragment.cs file is loaded first, and then a FrameLayout is loaded. In this project, the android:layout_weight attribute defines the relative width of each element. The TitlesFragment and the FrameLayout each display on 50 percent of the screen.
The TitlesFragment.cs file, shown in Listing 3, inherits from the Android.Support.V4.App.ListFragment class. This class is analogous to the ListFragment class available as a "regular fragment." The OnActivityCreated method that the class overrides is in charge of loading the appropriate user interface. The FragmentManager is exposed via Android.Support.V4.App.ListFragment class. This is different from the SupportingFragmentManager that is exposed via the FragmentActivity class.
The DetailsActivity.cs file, shown in Listing 4, is loaded when a user selects a Star Trek character and displays a picture and a quote. This code uses the SupportFragmentManager property of the FragmentActivity class. It loads the DetailsFragment. The OnCreate method creates an instance of the DetailsFragment, and then loads it via the FragmentManager that's exposed via the support libraries.
The DetailsFragment class, shown in Listing 5, inherits from Android.App.V4.App.Fragment class, which allows access to the fragment functionality.
The OnCreateView is used to create the necessary user interface. Notice that the user interface is created programmatically. The user interface could just as easily have been created by loading a layout file and then filling the values that way.
Different Emulators
Now that the code has been examined, let’s look at the output of the application. The list of Star Trek characters and detail view running in an Android 2.3.3 emulator are shown in Figures 4 and 5.
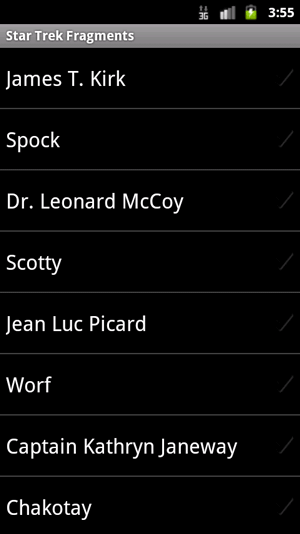 [Click on image for larger view.] |
Figure 4. List of Star Trek characters running on an Android 2.3.3 emulator. |
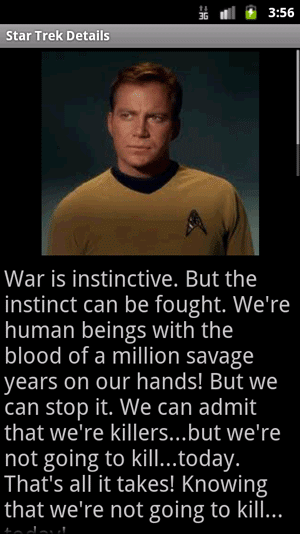 [Click on image for larger view.] |
Figure 5. Detail view of a Star Trek character taken from an Android 2.3.3 emulator. |
The list of Star Trek characters and detail view running in an Android 4.0.3 emulator are shown in Figures 6 and 7.
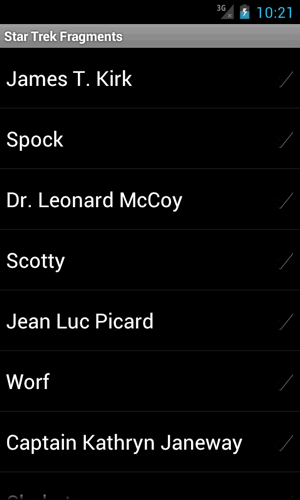 [Click on image for larger view.] |
Figure 6. List of Star Trek characters running on an Android 4.0.3 emulator. |
.
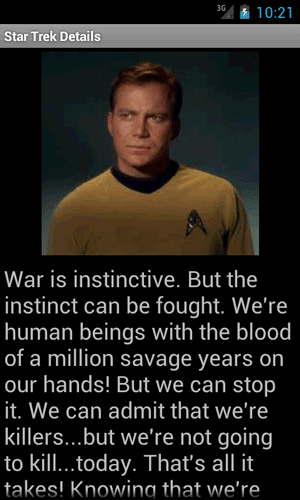 [Click on image for larger view.] |
Figure 7. Detail view of a Star Trek character taken from an Android 4.0.3 emulator. |
.
Notice that the only variance between the two displays is the slight difference in the way that the respective emulators are setup.
Finally, what happens when the application runs on a tablet device? Figure 8 shows the output of the Star Trek application in an Android 4.0.3 tablet emulator.
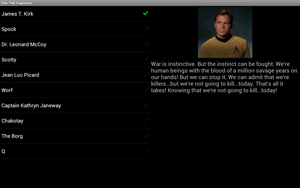 [Click on image for larger view.] |
Figure 8. Application running in an Android 4.0.3 tablet emulator. |
It's possible to write an application that runs on Android handsets, tablets, Android 2.x, 4.x, and to take advantage of many of the features on these platforms. Fragmentation? When people talk about fragmentation of the Android platform, I can’t help but wonder what they are talking about. After a little setup, Mono for Android can give you full support for the Android Support Package. The APIs are nearly the same between the Support Library and the most recent Android releases for Fragments.
About the Author
Wallace (Wally) B. McClure has authored books on iPhone programming with Mono/Monotouch, Android programming with Mono for Android, application architecture, ADO.NET, SQL Server and AJAX. He's a Microsoft MVP, an ASPInsider and a partner at Scalable Development Inc. He maintains a blog, and can be followed on Twitter.